1. 选择合适的解析器
在之前的学习中,我们使用html.parser
创建了BeautifulSoup对象。值得注意的是,BeautifulSoup支持多种解析器,每种解析器都有其独特的优势:
html.parser
:Python自带的解析器,速度适中,但容错能力一般。lxml
:解析速度快,容错能力强,需额外安装。html5lib
:提供最佳的容错性,但解析速度较慢,也需要安装。
让我们尝试使用不同的解析器来解析网页:
from bs4 import BeautifulSoup
import requests
url = "https://www.baidu.com"
headers = {
'user-agent': "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/128.0.0.0 Safari/537.36"
}
response = requests.get(url, headers=headers)
html_content = response.text
# 使用lxml解析器
soup_lxml = BeautifulSoup(html_content, 'lxml')
# 使用html5lib解析器
soup_html5 = BeautifulSoup(html_content, 'html5lib')
print(soup_lxml.title)
print(soup_html5.title)
小贴士:在处理不规范的HTML时,html5lib
表现最佳;而若HTML结构较为规范,使用lxml
能获得更高的性能。
2. 使用CSS选择器进行元素选择
我们可以通过find
和select
方法查找元素,其中select
方法支持所有CSS选择器,使我们能够像前端开发者一样精准地选择元素。
soup = BeautifulSoup(html_content, 'lxml')
# 选择所有class为'highlight'的<p>元素
highlights = soup.select('p.highlight')
# 选择id为'header'的元素内的所有<a>元素
header_links = soup.select('#header a')
# 选择所有含有'href'属性的<a>元素
all_links = soup.select('a[href]')
# 选择第一个<ul>中的所有<li>元素
first_list_items = soup.select('ul:first-of-type li')
for item in first_list_items:
print(item.text)
这种方式让我们仿佛回到了编写CSS的日子,尤其适合处理复杂的HTML结构。
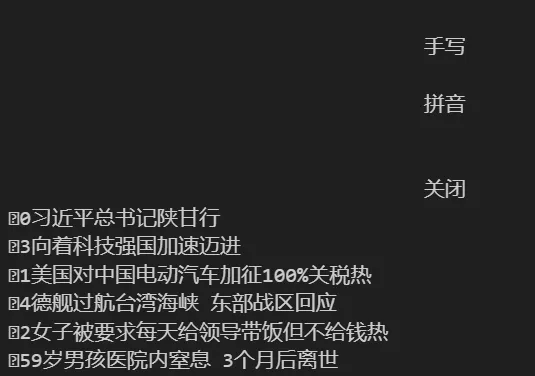
3. 处理标签属性
每个HTML标签都可能拥有多种属性,BeautifulSoup为我们提供了方便的方式来处理这些属性:
soup = BeautifulSoup(html_content, 'lxml')
# 获取一个标签的所有属性
link = soup.a
print(link.attrs)
# 获取特定属性的值
href = link.get('href')
print(href)
# 修改属性
link['class'] = 'new-class'
# 删除属性
del link['id']
print(link)
通过这种方式,我们可以轻松读取、修改和删除标签的属性。

4. 文档搜索技巧
有时,我们需要在文档中查找特定内容。BeautifulSoup的find_all
方法就像一把强大的放大镜,让我们能够快速找到所需的信息:
soup = BeautifulSoup(html_content, 'lxml')
# 找到所有包含"中国"的span
python_paragraphs = soup.find_all('span', string=lambda text: text is not None and '中国' in text)
print(python_paragraphs)
# 找到所有class包含"title-content-title"的元素
important_elements = soup.find_all(class_='title-content-title')
print(important_elements)
# 使用正则表达式查找所有以"hre"开头的属性
import re
for tag in soup.find_all(True): # True 用于查找所有标签
data_attrs = {key: value for key, value in tag.attrs.items() if re.match(r'^hre', key)}
if data_attrs:
print(f"Tag: {tag.name}, Data attributes: {data_attrs}")
使用字符串匹配、类查找以及正则表达式,BeautifulSoup为我们提供了强大的搜索能力!
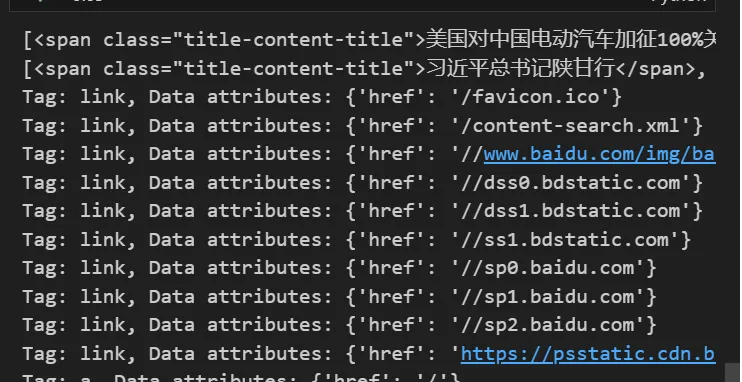
5. 解析部分文档
在某些情况下,我们可能只需要解析HTML文档的一部分。这时,SoupStrainer类将派上用场:
from bs4 import SoupStrainer
# 只解析<a>标签
only_a_tags = SoupStrainer("a")
soup = BeautifulSoup(html_content, 'lxml', parse_only=only_a_tags)
# 获取所有 <a> 标签的 href 属性
hrefs = [a['href'] for a in soup.find_all('a') if 'href' in a.attrs]
# 打印 href 列表
print(hrefs)
这种方法在处理大型HTML文档时特别有用,可以显著提高解析速度并减少内存使用。

6. 总结
在今天的学习中,我们深入探索了BeautifulSoup的许多高级特性:
- 不同解析器的选择与使用
- 使用CSS选择器精确定位元素
- 属性的获取、修改与删除
- 强大的文档搜索功能
- 部分文档解析技巧
这些功能使得BeautifulSoup在处理复杂HTML结构时更加游刃有余。记住,熟能生巧,多加练习才能真正掌握这些技巧!
感谢大家参与今天的Python学习之旅!希望你们能动手实践,如有问题欢迎在评论区提问。祝学习愉快,Python之路越走越宽广!
留言